Using the abstract keyword when declaring a class sets up a couple of things: first, as stated earlier, it means that the class itself cant be instantiated on its own in order to use it, it has to be extended by another class. class Person {. One cannot for example assert that x TypeScript Getter and Setter Property Example The base class initialized properties are initialized. Note that each member is public by default. TypeScript is a superset of JavaScript that introduces new features and helpful improvements to the language, including a powerful static typing system. abstract class BaseLogger {. Everything else extends it. It is a class that is inherited by multiple classes. The compiler needs this The Abstract Factory Pattern adds an abstraction layer over multiple other creational pattern implementations. Implement interfaces in TypeScript. Solution 1: To solve the problem, we can mark makeNoise with the abstract keyword. Construction is easy! We will discuss how to create date object in TypeScript. The resulting type of the property. Abstract classes are mainly for inheritance where other classes may derive from them.
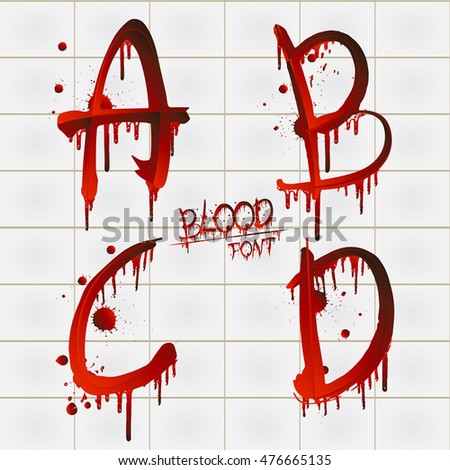
A NaiveBase class holds a private property, _myName and provides an get accessor to retrieve the value. Each of these type guards accept any Shape and return a boolean if the passed shape is of that type or not. Abstract classes cannot be instantiated. Were going to create a Node.js project and install TypeScript as a dependency. Children stores class constructors while InstanceType
They can only be extended. The current version of TypeScript 1.4 does't not have the notion of abstraction. The abstract classes allows us to create functionality which are implemented by sub classes (child classes). An abstract method/property is implemented in a derived class using the override keyword. Use the abstract keyword to indicate a class contains abstract methods or properties. The way above checks only the propertys existence.
Define the @special decorated properties of our class with a Special type. For example, add a name property to your Person class: In this example, you declare the property name with type string in addition to setting the property in the constructor. Note: In TypeScript, you can also declare the visibility of properties in a class to determine where the data can be accessed. A class declared with abstract keyword is known as an abstract class. About the author. The type variable K, which gets bound to each property in turn. Here is an example. All uni fied syntax trees are based off unist ( uni versal s yntax t ree). TypeScript - Abstract Class Define an abstract class in Typescript using the abstract keyword.
TypeScript . It shouldn't be instantiated directly. Typescript Conditional Operator Syntax: condition ?
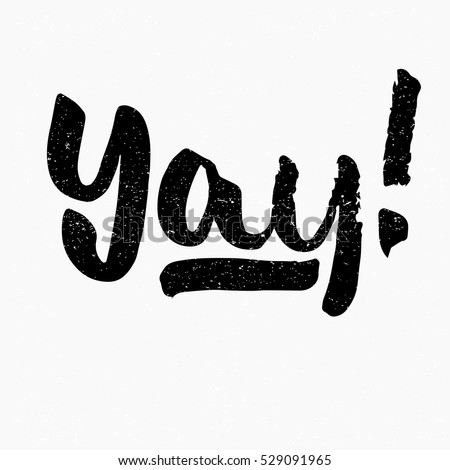
Example. Abstract classes # Two constructs can be abstract in TypeScript: An abstract class cant be instantiated.
Abstract classes cannot be instantiated, therefore the we cant make an object of the abstract class. TypeScript Array of Objects lets the user store multiple values in a single variable at once. You can think of it as looping through all properties one by one and applying some logic to each entry. These type guards can be combined with if statements to access properties only on a specific shape. 1. type IChildrenObj = {. An abstract class is different from an interface. TypeScript only knows at this point that we get a FilterItem back, and we cant instantiate FilterItem. public abstract double Area. JavaScript doesn't support interfaces so, as a JavaScript developer, you may or may not have experience with them. As for getters and setters., the type system does not differentiate between getter/setter and a property declaration. Follow @stemmlerjs. Abstract classes round out TypeScripts support for hierarchies of this nature. In this simple example, Keys is a hard-coded list of property names and the property type is always boolean, so this mapped type is equivalent to writing: We cannot create an instance of an abstract class. Although you will find examples of it also being used to return Builder, Prototypes, Singletons or other design pattern implementations. An abstract method/property has a signature in an abstract class. In TypeScript, an abstract class definition starts with abstract keyword.
About Projects. TypeScript provides a convenient way to define class members in the constructor, by adding a visibility modifiers to the parameter. He frequently publishes articles about Domain-Driven Design, software design and Advanced TypeScript & Node.js best practices for large-scale applications. The circle is Circle predicate narrows the type so the logic can branch accordingly. An abstract method has no implementation, only a type signature. An abstract class can have method(s) without body; in that case abstract keyword is used in the method signature.
abstract log (msg: string): void. Literal and Parent are more specific types which also extend Node. You can also use interfaces to define object types and this is the primary focus of this module. So far we have been doing this: Abstract Classes; Access Modifier: public, private and protected access; Both stacks and queues have storage and capacity properties as well as the size method.
You cant declare the class or members as non-overrideable, abstract or virtual (though interfaces provide much of the functionality that a virtual base class provides). An abstract class is declared by using the keyword abstract. In this way, you can deliver code that is type-safe, more reliable, and more representative of your business model. Code language: TypeScript (typescript) Typically, an abstract class contains one or more abstract methods. TypeScript is an open-source, object-oriented programing language, which is developed and maintained by Microsoft under the Apache 2 license. In TypeScript, a developer defines an abstract class using the abstract keyword. An abstract class can contain: One or multiple abstract methods. These methods are called abstract methods. Khalil Stemmler, Developer Advocate @ Apollo GraphQL . My proposal is in keeping with this behavior, except that abstract static and concrete static members exist on the first concrete subclass on down, not on the abstract class in which they were declared/defined. Syntax When considering how two properties with the same name relate to each other, TypeScript will only use the reading type (e.g. In an object destructuring pattern, shape: Shape means grab the property shape and redefine it locally as a variable named Shape.Likewise xPos: number creates a variable named number whose value is based on the parameters xPos.. Follow 2,498.
By adding types to your code, you can spot or avoid errors early and get rid of errors at compilation. May 10th, 2018 TypeScript compile-time inference assertions; May 9th, 2018 Interviewing in 2018; Jun 2nd, 2017 Management: Feel free to drop me an email at sufian@abstract.properties or send a tweet my way @sufianrhazi. TypeScript does not have "abstract" classes as such. abstract class Parent { abstract name: string; abstract get value (); abstract set value (v: number); } Typescript Abstract Class Property TypeScript is a strongly typed superset of JavaScript which compiles to plain JavaScript. Second, it allows the use of the abstract keyword on properties and methods inside of the class. The abstract keyword can be used both for classes and for abstract class methods.
An abstract method/property can't be static. An abstract method does not contain implementation. However, it's essential to know that you cannot instantiate an abstract class. In TypeScript, a developer defines an abstract class using the abstract keyword. Class Members Heres the most basic class - an empty one: class Point {} This class isnt very useful yet, so lets start adding some members. The example below has the BaseLogger, which is an abstract class that enforces derived classes to implement its abstract methods. We will also discuss how to add days to date in typescript, date format in typescript, typescript date now etc. Imagine that we are working on a Typescript project, and we have defined an interface with several fields using different types. It also defines the properties that are shared between all sub-classes, like value, valid, and dirty. An array of Objects is used to store a fixed-size sequential collection of elements of the same type. When declaring an abstract property (such as Area in this example), you simply indicate what property accessors are available, but do not implement them. 1. An abstract thing doesn't exist in reality e.g. Typescript Abstract Property 10/13/2020 A TypeScript Abstract class is a class which may have some unimplemented methods. Use the Special type to extract the decorated properties on the class.
TypeScript Abstract Class: Abstract class is a way of implementing 0 to 100% abstraction. Abstract Classes in TypeScript. abstract classes, which are classes that have partial implementation of a class and in which other classes can be derived from. Only its subclasses can if they are not abstract, themselves. The derived class initialized properties are initialized. However, it is possible to simulate the abstraction by combining overriding and exception. Use Cases Provide ability to list and use for reading an optional property in abstract class without limiting a way of its implementation in child classes. In TypeScript, you can use interfaces as you would in traditional object-oriented programming. The real difference comes when we consider our compiled JavaScript output. To use an abstract class, you must extend it.
Other concepts are Interfaces. Typescript supports object-oriented programming concepts using classes, interfaces, and abstract classes. Class is defined with the abstract keyword is called abstract classes. It is used to provide an abstraction. Instances for abstract classes are not created. Example Transforming properties we don't want to never This is the hardest part so well take it slow. An abstract class is a sealed class that cannot be instantiated directly. Copy. Since an abstract property must be extended in a sub class. TypeScript, of course, inherits this behavior and must abide by it. The most common use of abstract classes in TypeScript is to locate some common behavior to share within related subclasses. the type on the get accessor above). The two key characteristics of an abstract class in Typescript are: They can implement methods of their own. Fields Abstract classes have a major difference from regular JS classes -- they cannot be directly instantiated. It provides some of the shared behavior that all controls and groups of controls have, like running validators, calculating status, and resetting state. Before going with the detail, why would we want abstract with TypeScript? Create a new type that transforms all the decorated properties back to their original type but also adds a separate object with only the decorated properties. However, it's essential to know that you cannot instantiate an abstract class. mhegazy closed this as completed on Oct 2, 2015 Contributor Author That is why you call this and not super.. For the ngOnChanges side of things, I believe what's happening is your abstract class' method isn't TypeScript classes are even more powerful than JavaScript classes because they have access to the type system and new features such as member visibility, access modifiers, abstract classes, and much more. Abstract getter is NOT a part of this feature request, just shown for consistency. In this example, only a get accessor is available, so the property is read-only. Firstly, the reason you can't call super.somePropertyOfParent is because your abstract class isn't initialised separately from your derived class your derived class simply inherits all of the properties from the abstract class. We can use mapped types to create a new type based on the old. An abstract class is a class that includes both abstract and regular methods. 3. A getter is also called an accessor.
Unlike interface: An abstract class can give implementation details for its members. // name is a private member variable. As you can see, the subclass properties arent set until AFTER the base constructor is called. That means, this declaring the property as abstract does not change anything different from just declaring it. TypeScript - Abstract Class. TypeScript allows us to define an abstract class using keyword abstract. Abstract classes are mainly for inheritance where other classes may derive from them. It is a shorthand for creating member variables. We are always striving to enhance our usage of strong TypeScript types to make better software, and to make it easier to develop Grouparoo. (though you can override the property). 1. Typescript has the concept of a mapped type. TypeScript lets you augment an interface by simply declaring an interface with an identical name and new members. 4 types available - public, private, protected, Readonly public modifier: if the variable is declared without a modifier, the compiler treats it as public by default.This can be applied to variable declaration or constructor But because of structural typing, interfaces are somewhat weak. One of the best things in TypeScript, is automatic assignment of constructor parameters to the relevant property. Since abstract classes mix type information and actualy language (something that I try to avoid), a possible solution is to move to interfaces to define the actual type signature, and be able to create proper instances afterwards: An abstract class can also declare abstract member variables and also accessories such as setter and getter can be declared as abstract methods using set and get accessor typescript. If you are new to TypeScript, read my previous article on step by step tutorial on TypeScript. Strong types make it easy for team members to get quick validation about new code, and see hints and tips in their IDEs - a double win! Adopting the functional options pattern for class construction has other benefits, but in particular, it allows for the creation of a class object that may require asynchronous processes.The async function call can be added right into the class instantiation step, without needing a separate init() call or having to modify your established Abstract Factory defines an interface for creating all distinct products but leaves the actual product creation to concrete factory classes. Mercedes car does exist. An abstract class in TypeScript is defined by the abstract keyword.
In this case, typeof needs to be used to check the data type. To demonstrate, let's imagine that we are building a "pluck" function:
Decorators. Classes, constructor functions and this keyword, private and public, initialization, readonly, inheritance, overriding, protected, getters & setters, static, abstract and singletons! It provides some of the shared behavior that all controls and groups of controls have, like running validators, calculating status, and resetting state. Its meant to be derived by other classes and not to be instantiated directly. Properties can also be marked as readonly Sounds abstract. We can't create an instance of an abstract class.
Keep in mind, this isnt a pattern thats limited to classes. This is like an application-level schema. To use an abstract class, you must extend it. The compiler might help you providing autocomplete for properties of Person but it will not complain if you miss any properties.
TypeScript 2.8's conditional types can be used to create compile-time inference assertions, which can be used to write tests that verify the behavior of TypeScript's inference on your API. At Grouparoo, we use a lot of TypeScript. That will enforce derived classes to implement this method on their An abstract method/property has an implementation in a derived class. Luckily in Typescript, we can use abstract classes. A declaration file is a special type of file used by the TypeScript compiler. TypeScript doesn't support multiple inheritance (although your class can implement multiple interfaces). Abstract class in TypeScript. Its just a relational map generated and placed over your JavaScript code. An abstract class looks and feels like a standard class with a key exception: abstract classes may never be instantiated. We can not create objects of an abstract class. Property 'instantiatedAt' has no initializer and is not definitely assigned in the constructor. An abstract class can also declare abstract member variables and also accessories such as setter and getter can be declared as abstract methods using set and get accessor typescript. Types which are globally included in TypeScript. ; Second, add the getHeadcount() static method that returns the value of the headcount static property.
It also defines the properties that are shared between all sub-classes, like value, valid, and dirty. In the example above, the methods object in the argument to makeObject has a contextual type that includes ThisType
In typescript, There are different types of accessor types applied to instance members or variables or properties and methods. Using mapping modifiers, you can remove optional attributes.. readonly Properties. expr1 : expr2. TypeScript also has a shortcut for writing properties that have the same name as the parameters passed to the constructor. To begin with, in simple terms, think if it as a Factory that can return Factories. We need to initialize a TypeScript project as well: npx tsc --init TypeScript also comes with fancier ways of achieving inheritance. An abstract class allows to marks its members methods as private and protected. It only defines the signature of the method without including the method body. In TypeScript, classes or interfaces can be used to create models to represent what our documents will look like. An abstract method/property is implicitly a virtual method/property. Classes can define what properties an object should have, as well as what data type those properties should be. Given two types, say Colorful and Circle, you can combine set properties in interesting ways via union and intersection.. With union types, narrowing is necessary via a type predicate. Photo by niko photos on Unsplash. If two interfaces have the same properties but one of the data types is different, we somehow need to differentiate them. Method Overriding is useful when sub class wants to modify the behavior of super class for certain tasks. Such problem occured in a real project because of migration from TS3 to TS4. abstract class Main {run() {const myObject = { a: 2, b: 4 }; console.log(`Dot Notation (good): ${myObject.a}`); console.log(`Bracket Notation (bad): ${myObject['a']}`);}} Main.run();The only use case I can justify using the object bracket notation Abstract class in TypeScript. (2564) This is an additional TypeScript safety check to ensure that the correct properties are present upon class instantiation. TypeScript 4.3 also supports constructor parameters on abstract classes. the type is going to always have the property. It shouldn't be instantiated directly.
public constructor (private name: string) {} public getName (): string {. 2. The types provided by uni st are abstract interfaces. Lets create a new NativeScript app using the TypeScript template. ; To call a static method, you use the A setter method updates the propertys value. 2. One or numerous property declarations. TypeScript makes inheritance easy by letting us define abstract classes where some implementation is done by the abstract and others are done in the subclass that extends the abstract class. We also have abstract methods that subclasses can implement.