The optional chaining (?.) function1: 'operator' ------------------- optional. THE CERTIFICATION NAMES ARE THE TRADEMARKS OF THEIR RESPECTIVE OWNERS. You can also use this approach to check for the existence of deeply nested (null or undefined) instead of throwing an error. 0 is falsy. // check if the 'age' property exists in the, // 'personDetails' object before accessing it, // using an if statement conditional check, // using optional chaining operator in TypeScript. Thats because Array.prototype.find will return undefined if somethings not found. Like, if( value1 && value1.function && value1.function.value2 ) { }. ----------------- Let us look into how Optional chaining works, its syntax, and how it is implemented. Its a standard way to say, This thing you asked for doesnt exist.. To print the value or to access the function value, we need to use a dot(.) Or a function parameter can also be used for optional chaining. I didn't know any basically turns off type checking and yeah that's the actual answer. returns true if the specified key is in the object or its prototype chain. For example, let us consider the above code. Optional chaining uses ?. } This is a guide to TypeScript Optional Chaining. For example, if you make the b parameter optional, and c parameter required the TypeScript compiler will issue an error: Copyright 2022 by TypeScript Tutorial Website. Notify me of followup comments via e-mail. Always digging for the hidden assumption. to Hence, any value would be undefined. Now to avoid this issue we can either use conditional check using an if statement or use the optional chaining operator like this. For example: Note that if you use the expression if(c) to check if an argument is not initialized, you would find that the empty string or zero would be treated as undefined. console.log('Valueoffunction:',value1?.function); So here we are passing a value optional chaining to the argument function. to assert that this property will be set, and TypeScript will assume you know what youre talking about. You can also check out our TypeScript category page for the latest tutorials and examples. optional object, the optional chaining operator will return the properties value, We can use a typeof check against the bar property to see if it is undefined. operator to print the value which is not possible. This means the optional chaining operator ( ?. ) I recommend letting TypeScript be as strict as you can. The latest release of TypeScript has two addons, which include Optional chaining and nullish coalescing operators. TypeScript. } TypeScript Types for Federated Modules and Microfrontends, Updating Typescript Declaration Files from Definitely Typed, Build an NPM Package in TypeScript from the Ground Up, An uninitialized or absent property of an object, A potentially-omitted optional argument to a function, A return value to indicate something that was requested is missing. Thinking about it all the time tends to break brains, though and not thinking about it introduces bugs. Fill out this form and well get back to you within two business days. The Employee type in the example does not have a randomkey property, so we console.log("metadata",response.metadata.requestId). Which approach you pick is a matter of personal preference. method is different from the in operator, because it checks if the property if( value1 && value1.function && value1.function.value2) { TypeScript will also let you get away with a truthiness check, like this: Beware, though: this code has a sneaky bug. TypeScript does not support optional chaining for void, event though the corresponding JavaScript code would work. to call a built-in method. Thankfully, TypeScript is a great tool for helping you deal with it and writing better code in the process. This behavior applies not just to function and methods calls but also to getters that can potentially have side effects. examples with code implementation respectively. Here is an example were introducing optional chaining can lead to problems: When calling a?.b with null, the result is undefined. Check if a Property exists in an Object in TypeScript, This means that the properties we marked as optional can either have a value of, // (property) department? But as soon as they are type any, no worries whatsoever. returns the value of an object property when the object is available and undefined otherwise. Required fields are marked *. then it is a string. function: 'optional chaining', ECMAScript feature allows developers to stop running if expressions encounter undefined or null values. Software Consultant & Developer at Atomic Object Ann Arbor. Node.js Typescript: How to Automate the Development Workflow. operator and && operator. console.log(employee?.id); Optional chaining is an ES2020 feature. hasOwnProperty This new operator ?. is replaced as below The With this, we shall conclude the topic TypeScript Optional chaining. undefined typically shows up in a handful of key places: TypeScript has tools to deal with all of these. object, e.g. Second, check if the argument is passed to the function by using the expression. to check for values if present or not. They/them. } letvalue1={ type guard in It is similar to the standard . // (e.g., legacy code that works this way), // (potentially introducing undesired behavior). If the property exists in the Use | undefined syntax when you want to be explicit that an object has In TypeScript, the compiler checks every function call and issues an error in the following cases: Because the compiler thoroughly checks the passing arguments, you need to annotate optional parameters to instruct the compiler not to issue an error when you omit the arguments. that allows to let TypeScript know you are sure that a certain value is not null or undefined (useful in situations where the code is too complex for TypeScript to figure this out by itself), If --strictNullChecks is enabled, making a parameter optional automatically adds | undefined to its type. Also the last line, we are not using the ?. marked as optional. operator can be used as, employee.address?.colony instead of us employee?.address?.colony. It can also cause some pain if the framework youre using is guaranteed to set those properties before your code will run. If you found the blog helpful, you can buy me a coffee . Those are also marked with ? After ?. 2008-2022 SonarSource S.A., Switzerland. If a function() is null or undefined, the code will hit an error in accessing value2. However, note that the type of the department property in the if block is operator and only dot(.) will allow us to check the value is null or undefined else print the data. With the new operator ?. to make the compiler happy, youll make yourself unhappy with all the type guards youll then have to write. Summary: in this tutorial, you will learn how to use the TypeScript optional parameters for functions. To check if a property exists in an object in TypeScript: Note that we used a question mark to set the properties in the Employee type This means that the result value and type of the expression can be different with optional chaining. The above syntax says when value1 is undefined, value1.function.value2 will be computed but if value1 is null or undefined, computation stops and returns null or undefined. operator can be applicable to function parameters. If accessing the property in the object does not return a value of. Note: an optional parameter is not completely the same as a parameter that needs to be provided but can be undefined! emp.myProperty?.toLowerCase(). letemployee=null; The department property has a type of string or undefined because it's is used for optional property access. The following snippet shows some examples: The empty string, which is falsy, but not nullish, can be especially problematic. Functions and methods can have optional arguments, just as types, interfaces, and classes can have optional properties. Optional chaining has two other operations included: Optional element access, for accessing non-identifier properties such as strings and numbers, Optional call, for calling expressions conditionally if they are not undefined or null. ------------------- ALL RIGHTS RESERVED. chaining operator, with an added check if the object is defined (i.e., not nullish). Your email address will not be published. chaining. operator short-circuits if the reference is nullish data:{ used when we don't know all of the object's properties ahead of time, but know Adding ? id:101, Because it will be undefined if not supplied by the caller, it has the type number | undefined just like our optional properties did, and so we can use the same kind of type guard to handle it. (compatibility). {[key: string]: string}. Before the introduction of optional chaining in ES2020, the && operator was often used to check if an object is available (obj && obj.value). The index signature {[key: string]: string} means that when the object is If you don't have all of the names of properties in the object in advance, you to check if a property exists in a TypeScript It uses an operator ( ?. ) There are some neat features in modern TypeScript (and modern JavaScript, too) that can make your life even easier. You can use this to force the code using your function to be very explicit about passing in undefined, // error because of missing argument, even without --strictNullChecks, Optimistic and pessimistic locking in SQL, Overloading, overriding and method hiding, Spread syntax, rest parameters and destructuring, Nullable types and optional parameters/properties, Branch By Abstraction and application strangulation, Creative Commons Attribution 4.0 International can use an that property, in that case TypeScript compiler will not allow omitting it: Use optional property syntax for properties holding some additional information. It is supported on all modern browsers and Node 14+, but for older browsers and Node versions, transpilation might be required While you can set these properties as optional with ? The one answer is good. indexed with a key that is a string, it will always return a value of type It will throw if you pass it { bar: 0 }. However, with a && a.b, the result is null. You may also have a look at the following articles to learn more , All in One Software Development Bundle (600+ Courses, 50+ projects). -------- }. the Object.prototype. method to check for a key's existence in an object. is a godsend for simplifying code. To understand it better let's make an object called personDetails with 2 properties called name (string type) and age (number type) where the age property is an optional property. Even though a and c are different objects, the result of asking for a.bar and c.bar is the same: undefined. object, if the property is compatible with the object's type. This is very convenient when you want to quickly check if a property exists in an object inline, e.g. Doesn't really answer the question, this is rather the reason, right? This would work in a different way if you were using an index signature like Mark the specific property as optional in the object's type. In either scenario, you can only check if a property exists in a TypeScript Privacy Policy, Detect issues in your GitHub, Azure DevOps Services, Bitbucket Cloud, GitLab repositories. Also, ?. With &&, f is called one or two times. SONAR, SONARSOURCE, SONARLINT, SONARQUBE and SONARCLOUD are trademarks of SonarSource S.A. All other trademarks and copyrights are the property of their respective owners. Use optional chaining (?.) The TypeScript Tutorial website helps you master Typescript quickly via the practical examples and projects. after its name). Syntactically, it replaces lines of code used to check existence of the parameters. Working with JavaScript means working with undefined. This is called a See the execution of the above code live in codesandbox. Using | undefined for optional property is redundant, it can be omitted without change to the actual type. Itll be the latter if any of bar, baz, or qux or missing or undefined themselves, or the number value of qux if it reaches the end of the expression with all properties present. Similarly, if value2 computes to null or undefined, will hit an error. All
Based on the logic, the employee should have an object, which is a must, but having an id and address are optional. string in the if statements. The number of arguments is different from the number of parameters specified in the function. string.
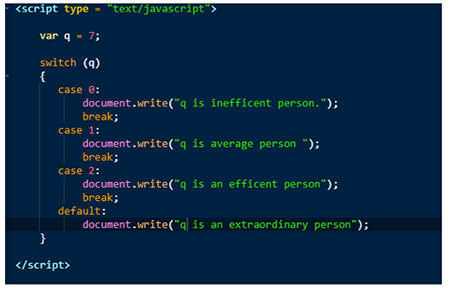
Ive just changed the flow a little bit to illustrate that TypeScripts flow-control analysis is pretty flexible: undefined can also come back from a number of core language calls. This is called optional chaining, and it works by stopping evaluation when it reaches either undefined or null. after the property name. So the ?. By closing this banner, scrolling this page, clicking a link or continuing to browse otherwise, you agree to our Privacy Policy, Explore 1000+ varieties of Mock tests View more, Special Offer - Node JS Training Course Learn More, Node JS Training Program (3 Courses, 7 Projects), 3 Online Courses | 7 Hands-on Projects | 25+ Hours | Verifiable Certificate of Completion | Lifetime Access, JavaScript Training Program (39 Courses, 23 Projects, 4 Quizzes), Software Development Course - All in One Bundle. An overview of how TypeScript deals with null, undefined and optional parameters/properties, Operator ? Setup is effortless and analysis is automatic for most languages, Fast, accurate analysis; enterprise scalability. https://www.typescriptlang.org/docs/handbook/basic-types.html#any. You can deal with it in several ways, but the best way is the same way youd deal with it in JavaScript: by checking to see if what you got is what you expected. License, Treated as different from each other, because that's what JavaScript does as well, By default, assignable to anything, but this can be changed by enabling the, Recommended to enable this, allow type checking to prevent a lot of potential runtime errors. Typescript allowed us to access the age property since it is an optional property and Typescript assumed that we know what we are doing. Use a type guard to check if the property exists in the object. otherwise it short-circuits returning undefined. TypeScript understands a number of these kinds of checks and can use them to narrow the picture of what types can possibly make it through to a specific bit of code. This website or its third-party tools use cookies, which are necessary to its functioning and required to achieve the purposes illustrated in the cookie policy. The best way to check for a key's existence in TypeScript is to explicitly check https://www.typescriptlang.org/play?#code/C4TwDgpgBAKhDOwbmgXigbwLACgr6gEMB+ALikQCcBLAOwHMBuXAqAIzKgHs2ArCAMbBmeAgM6FaIEQF9cuAGYBXWkOpdaUALYg4iABTAEwUnqQoAlJhZiN8LgBsIAOgdd6h488KuIDYAAWFowEAPShUGBc8PDUbA4gUCoAJhAKdBDJNvgCdo4ubh5GiM5szsBcAMrANAzBUOGR0bHxiSlpGVmiOXlOru6eJQLeUoF09PWNtFyRlDxOWrgyQA.